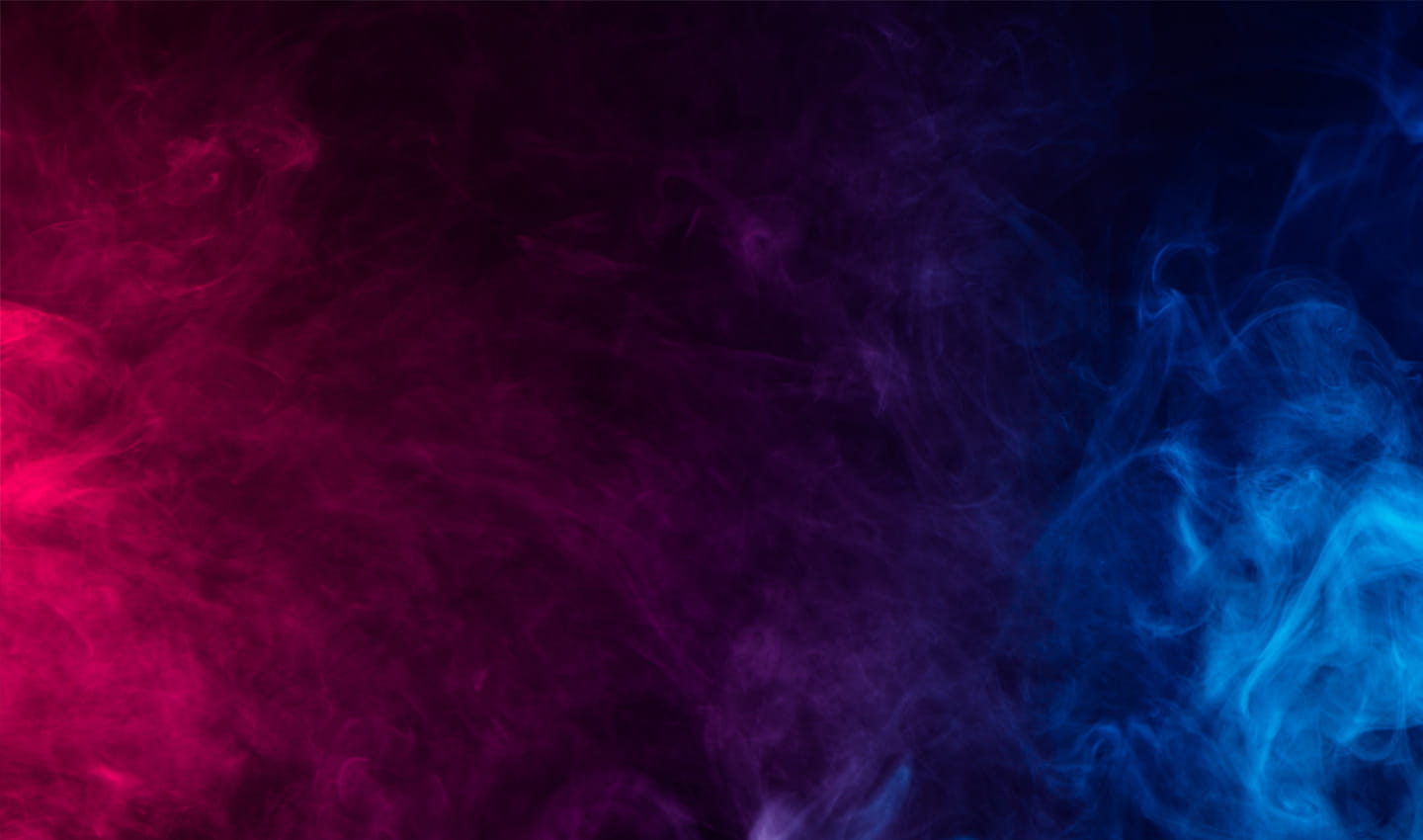
andreimerlescu.com is available for purchase at NameLiquidate.com
This expired domain is available for purchase at NameLiquidate.com!
The domain you are buying is delivered upon purchase.
Buy with confidence. Your purchase is secured by Epik.
Free WHOIS privacy, free forwarding, 24/7 Support are all standard.